[Next.js 13.5.2] [App Router] [Route Handler] Request Payload Validation
Unanswered
Blue swimming crab posted this in #help-forum
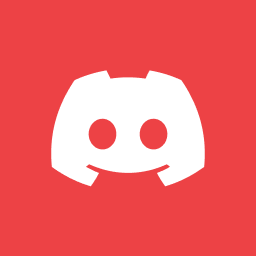
Blue swimming crabOP
schema.prisma
:enum BoardType {
Public
Restricted
Private
}
enum BoardStatus {
Active
Deactivated
Deleted
}
model Board {
id BigInt @id @default(autoincrement()) @db.BigInt
boardType BoardType
name String @unique
allowPosts Boolean @default(true)
status BoardStatus
createdAt DateTime @default(now())
modifiedAt DateTime?
deletedAt DateTime?
@@map("boards")
}
app/boards/route.ts
:import { PrismaClient } from '@prisma/client';
import { NextRequest, NextResponse } from 'next/server';
const prisma = new PrismaClient();
export async function POST(req: NextRequest) {
try {
const data = await req.json();
const board = await prisma.board.create({ data });
return NextResponse.json(board, { status: 201 });
} catch (err) {
return NextResponse.json(err, { status: 400 });
}
}
I have a post request handler
POST
, as you can see in the code above. I want to validate the request payload.If the payload doesn't contain the correct
BaordType
, BoardStatus
, required fields, or invalid type
of values, I want to immediately return with 400 bad request.I can achieve this by hardcoding the validation, but is there a cleaner way to do this with a library? Is
zod
appropriate for this situation?