Image component from next/image and Tailwind CSS
Unanswered
Red-billed Tropicbird posted this in #help-forum
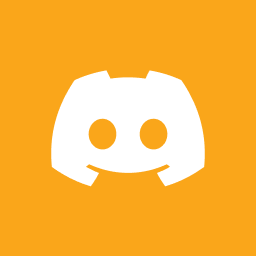
Red-billed TropicbirdOP
Hi,
I want to use the Image component from Next/Image, and style it with Tailwind CS. But it does not seem to be working properly because it expects me to either specify the
Using the
I want to use the Image component from Next/Image, and style it with Tailwind CS. But it does not seem to be working properly because it expects me to either specify the
width
and height
if I use the source directly in the image component, or it will figure out the size automatically if I import it in the file on top as seen below: import Image from 'next/image'
import adamwathan from '../public/adamwathan.jpeg'
export default function Home() {
return (
<div className='bg-gray-700 text-white min-h-screen flex items-center justify-center'>
<Image src={adamwathan} alt='test' className='rounded-full w-10 h-10' />
</div>
)
}
Using the
w-10 h-10
on className with Tailwind CSS yields no change.66 Replies

First, what physical size is the image?
Second, what happens if you remove the flex class from its parent?
Second, what happens if you remove the flex class from its parent?

@Morgan First, what physical size is the image?
Second, what happens if you remove the flex class from its parent?
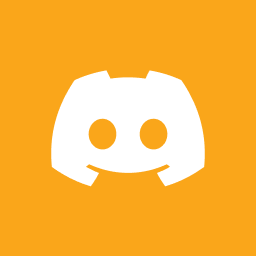
Red-billed TropicbirdOP
460x460

You might need to set a max-width on the image

@Morgan You might need to set a max-width on the image
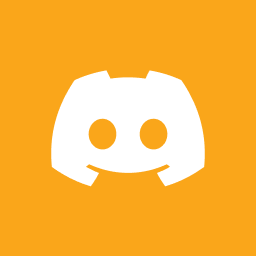
Red-billed TropicbirdOP
Removng flex just moves the image to the left top corner

Ok. Is there one already? Eg max-width: 100%?
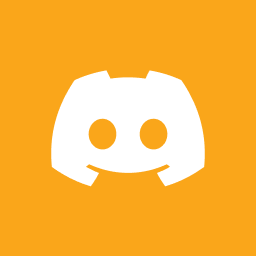
Red-billed TropicbirdOP
instead of being center

@Morgan Ok. Is there one already? Eg max-width: 100%?
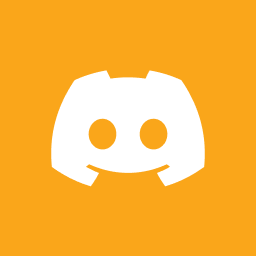
Red-billed TropicbirdOP
Nope, I only wrote what is in the code
Should I try max-width?

Always use max-width: 100% height: auto as defaults on images otherwise they overflow.

@Morgan Always use max-width: 100% height: auto as defaults on images otherwise they overflow.
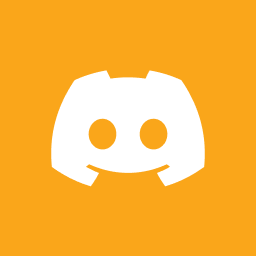
Red-billed TropicbirdOP
e.g. as part of tailwind className?

@!isColonel Click to see attachment

Required Props

@!isColonel **Required Props**
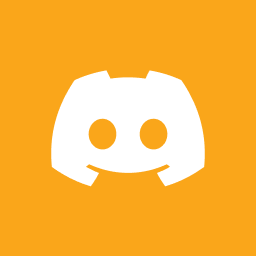
Red-billed TropicbirdOP
Yea they are required, but if that is the case then I will just regular old
img
html tagsince I want to style with tailwind
export default function Home() {
return (
<div className='bg-gray-700 text-white min-h-screen flex items-center justify-center'>
<img src='/adamwathan.jpeg' alt='test' className='rounded-full w-10 h-10' />
</div>
)
}
This works flawlessly
img

Can still style img tag but u loose the quality and priority props which help make image more better

It’s the actual img element you need to apply max-width on

@!isColonel Can still style img tag but u loose the quality and priority props which help make image more better
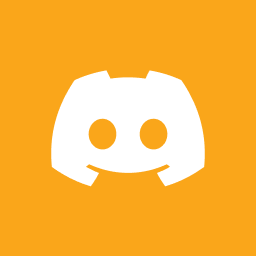
Red-billed TropicbirdOP
So you're saying I should just use the
Image
with the required props and forget about using the tailwind w
and h
styles?:)(but use everything else from Tailwind)
import Image from 'next/image'
import adamwathan from '../public/adamwathan.jpeg'
export default function Home() {
return (
<div className='bg-gray-700 text-white min-h-screen flex items-center justify-center'>
<Image src={adamwathan} alt='test' width={40} height={40} className='rounded-full' />
</div>
)
}
This works
I guess thats just how people do it?
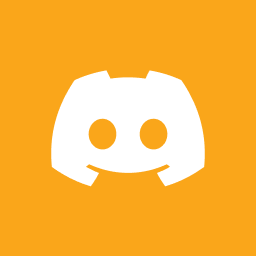
@Red-billed Tropicbird So you're saying I should just use the `Image` with the required props and forget about using the tailwind `w` and `h` styles?:)

Use what u want. If image allows u to do what u want than use that but Image from next js requires those props. U can use Image and use the style={{}} and make it differently I think but like I said if priority and quality props dont matter just leavit
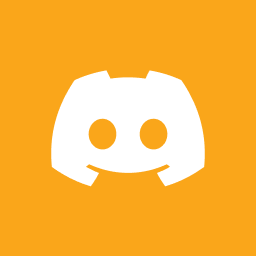
Red-billed TropicbirdOP
seems weird to have styles both as its props and inside classname

@!isColonel Use what u want. If image allows u to do what u want than use that but Image from next js requires those props. U can use Image and use the style={{}} and make it differently I think but like I said if priority and quality props dont matter just leavit
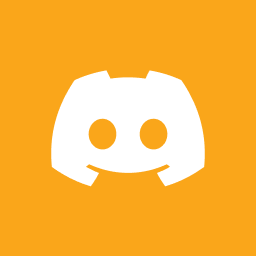
Red-billed TropicbirdOP
I prefer to learn the 'correct' nextjs way, which is the Image component
but I was just a bit confused as to why it had width and height as required props
seems like you answered
its probably due to making the quality and priority better

Well its just the way nextjs is.

I thought width and height aren’t required when importing the image?
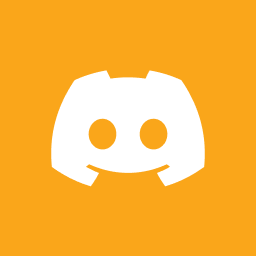
Red-billed TropicbirdOP
so I will use Image component with with and height required props, and tailwind CSS className for everything else in styles

@Morgan I thought width and height aren’t required when importing the image?
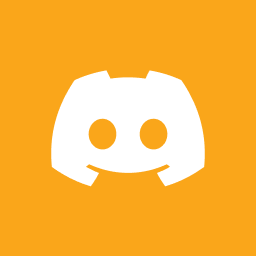
Red-billed TropicbirdOP
It isn't
but if one wants to specify something
thats the way to do it, it seems

So this doesn’t make sense.

You using ts or js?

@!isColonel You using ts or js?
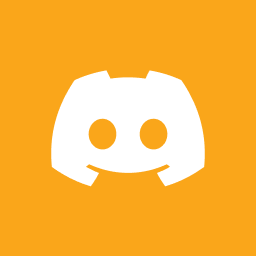
Red-billed TropicbirdOP
TS

I think ive gotten away with just using width prop on js but ts is way strict.

@Morgan So this doesn’t make sense.
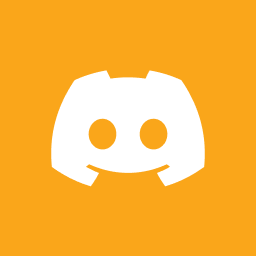
Red-billed TropicbirdOP
import Image from 'next/image'
import adamwathan from '../public/adamwathan.jpeg'
export default function Home() {
return (
<div className='bg-gray-700 text-white min-h-screen flex items-center justify-center'>
<Image src={adamwathan} alt='test' className='rounded-full' />
</div>
)
}
I can do it without width and height when I import it
but I specified
width={40} height={40}
to make it smaller.The default is huge (e.g. original image size)

Well that makes sense as the image is massive
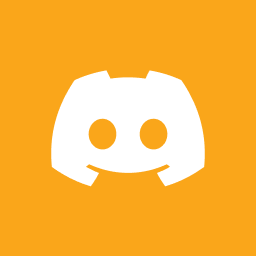
Red-billed TropicbirdOP
I wanted to resize it with tailwind css className, and I couldn't
w-10 and h-10 does nothing
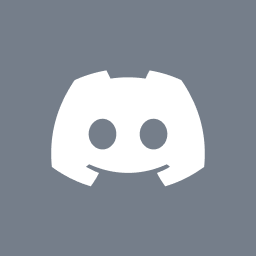
Cairn Terrier
Wrap it in a div and make it fill that maybe?
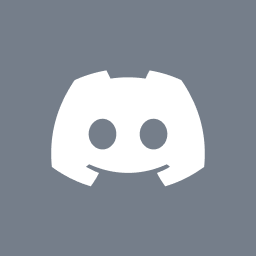
@Cairn Terrier Wrap it in a div and make it fill that maybe?
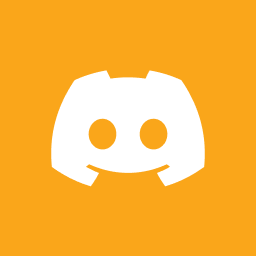
Red-billed TropicbirdOP
seems like more work than just abandoning
w
and h
tailwind css styles in className, and just use width={40} height={40}
props as part of the Image component itself
Not worth the hassle. But if you’re interested to know more, check what element the class is applied to.

I did this for one of mine
<Image
priority
quality={100}
width={300}
height={300}
className="p-1 rounded-full ring-2 ring-sky-300 w-1/2 md:w-full md:h-full "
style={{
width: "205px",
height: "205px",
objectFit: "cover",
}}
src={Me}
alt="Developer profile image"
/>

@!isColonel I did this for one of mine
js
<Image
priority
quality={100}
width={300}
height={300}
className="p-1 rounded-full ring-2 ring-sky-300 w-1/2 md:w-full md:h-full "
style={{
width: "205px",
height: "205px",
objectFit: "cover",
}}
src={Me}
alt="Developer profile image"
/>
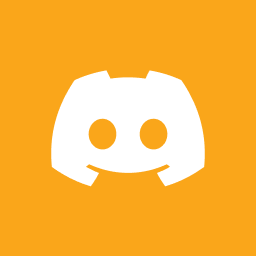
Red-billed TropicbirdOP
does your image resizing work in the
className

Made it smaller with style prop idk 🤷â€â™‚ï¸. Anyway ya
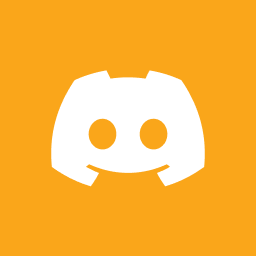
Red-billed TropicbirdOP
even after setting width and height props?

I dont think so. I can remove them cuz i style it with uh style prop. You trying to make it bigger and smaller depending on screen size?

@!isColonel I dont think so. I can remove them cuz i style it with uh style prop. You trying to make it bigger and smaller depending on screen size?
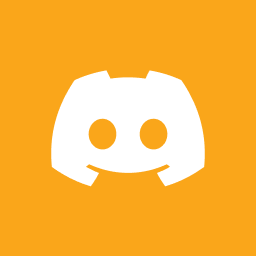
Red-billed TropicbirdOP
Im just trying to make it smaller in general

Tried a smaller number? In width and height prop?

@Morgan But then overriding w-full

Ya imma remove

@!isColonel Tried a smaller number? In width and height prop?
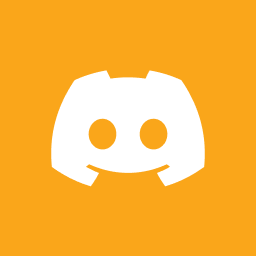
Red-billed TropicbirdOP
Yes that work
but I wanted to do in classname using tailwind css

Lol
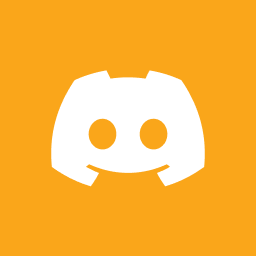
Red-billed TropicbirdOP
but I got it now
I have to use the required props

In my experience, the more u let next js do for you, cleaner the code will be