Component sizes too small? Unsure on best practices for App Router
Unanswered
American black bear posted this in #help-forum
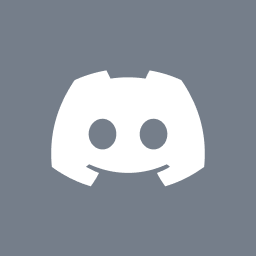
American black bearOP
I'm using the App Router with next.js
From my understanding, it's a good idea to keep as much content as possible SSR
If I have a component which needs to be a client component, should I make it as small as possible?
Here are two examples:
vs
is the second option preferred? I'm wondering if moving those buttons into their own components is a bit overkill
From my understanding, it's a good idea to keep as much content as possible SSR
If I have a component which needs to be a client component, should I make it as small as possible?
Here are two examples:
"use client";
import { signIn, signOut, useSession } from 'next-auth/react';
import { FaDiscord } from 'react-icons/fa';
export default function DiscordLogin() {
const session = useSession();
console.log('session', session);
if (!session) {
<button
className="max-w-[14rem] bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded flex items-center"
onClick={() => signIn('discord')}
>
<FaDiscord className="mr-2" />
Sign in with Discord
</button>
}
return <>
<button
className="max-w-[20rem] bg-red-500 hover:bg-red-700 text-white font-bold py-2 px-4 rounded flex items-center"
onClick={() => signOut()}
>
<FaDiscord className="mr-2" />
Signed in as {session.data?.user?.name}. Sign out
</button>
</>;
}
vs
import SignInButton from './SignInButton';
import SignOutButton from './SignOutButton';
import { getServerSession } from 'next-auth';
import { authOptions } from '@/app/api/auth/[...nextauth]/route';
export default async function DiscordLogin() {
const session = await getServerSession(authOptions);
console.log('session', session);
if (!session) {
return <SignInButton />
}
return <SignOutButton username={session.user!.name as string} />
}
is the second option preferred? I'm wondering if moving those buttons into their own components is a bit overkill
1 Reply
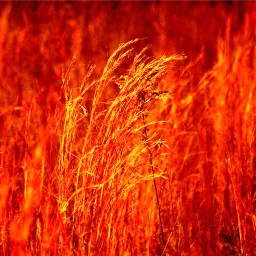
this is a few days later, but SSR is always done, even for client components (hooks aren't done, so you have a point in this example)... But server components gives better UX to have as much server rendered as possible (less layout changes).
(and your first example probably is missing a return after the if)
(and your first example probably is missing a return after the if)