How do I resolve webpack loaders error in Next.js 13.4.8 when I try to add a background video?
Answered
Western thatching ant posted this in #help-forum
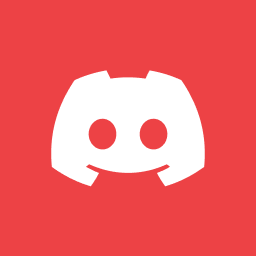
Western thatching antOP
I am creating a webpage with Next.js 13.4.8 and when I try to add a background video to the page, I get this error:
The code for the component that contains the background video looks like this:
How can I resolve this error?
Failed to compile
./public/homeFeatureSectionVideo.mp4
Module parse failed: Unexpected character '' (1:0)
You may need an appropriate loader to handle this file type, currently no loaders are configured to process this file. See https://webpack.js.org/concepts#loaders
(Source code omitted for this binary file)
This error occurred during the build process and can only be dismissed by fixing the error.
The code for the component that contains the background video looks like this:
"use client"
import React, { useState, useEffect } from 'react'
import './homeFeature.css'
import homeFeatureSectionVideo from '../../public/homeFeatureSectionVideo.mp4'
export const HomeFeature = () => {
const [hasWindow, setHasWindow] = useState(false)
useEffect(() => {
if (typeof window !== 'undefined') {
setHasWindow(true)
}
}, [])
return (
<>
{hasWindow &&
<div className="homefeature">
<video src={homeFeatureSectionVideo} autoPlay muted loop className='video-bg' />
<div className='bg-overlay'></div>
<div className="feature-text">
<h1>George Muu & Associates</h1>
<p>The premier audit firm in Kenya</p>
</div>
<div className="feature-btn">Talk to Us</div>
</div>
}
</>
)
}
How can I resolve this error?
Answered by Rafael Almeida
files in the
public
folder are served from the root of the web server, the src
prop takes an URL path to a resource, not a filename path. in your case you would use it like this:<video src="/homeFeatureSectionVideo.mp4" autoPlay muted loop className='video-bg' />
5 Replies
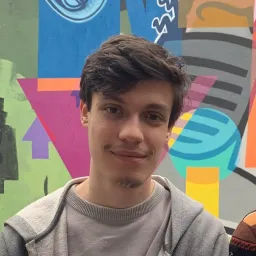
Rafael Almeida
next doesn't have any webpack loaders for
.mp4
files, if you want to use the import
syntax you need to add one yourself. alternatively, you can move the .mp4
file to the public
folder and reference it with an URL path: <video src="/your-video.mp4" />
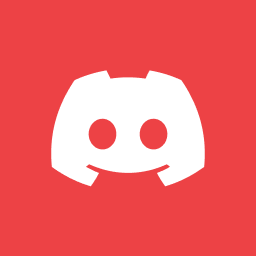
Western thatching antOP
I have changed the code to this, in line with the guideline you gave:
The video homeFeatureSectionVideo.mp4 is already in the public folder...but I am getting this error:
How can I resolve this?
"use client"
import React, { useState, useEffect } from 'react'
import './homeFeature.css'
export const HomeFeature = () => {
const [hasWindow, setHasWindow] = useState(false)
useEffect(() => {
if (typeof window !== 'undefined') {
setHasWindow(true)
}
}, [])
return (
<>
{hasWindow &&
<div className="homefeature">
<video src="../../public/homeFeatureSectionVideo.mp4" autoPlay muted loop className='video-bg' />
<div className='bg-overlay'></div>
<div className="feature-text">
<h1>George Muu & Associates</h1>
<p>The premier audit firm in Kenya</p>
</div>
<div className="feature-btn">Talk to Us</div>
</div>
}
</>
)
}
The video homeFeatureSectionVideo.mp4 is already in the public folder...but I am getting this error:
homeFeatureSectionVideo.mp4:1 GET http://localhost:3002/public/homeFeatureSectionVideo.mp4 404 (Not Found)
How can I resolve this?
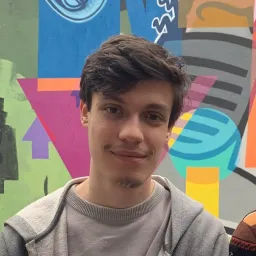
Rafael Almeida
files in the
public
folder are served from the root of the web server, the src
prop takes an URL path to a resource, not a filename path. in your case you would use it like this:<video src="/homeFeatureSectionVideo.mp4" autoPlay muted loop className='video-bg' />
Answer
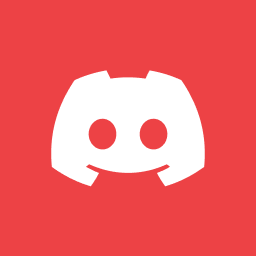
Western thatching antOP
Oh man, thanks. It now works!