NextApiRequest req.body wrong format
Answered
Atlantic herring posted this in #help-forum
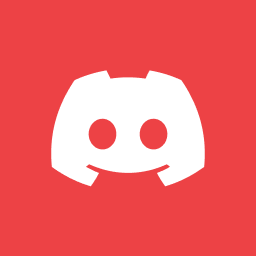
Atlantic herringOP
I have this curl POST request:
curl -X POST http://localhost:3000/api/test -H 'Content-Type:application/json' --data '{"username":"xyz","password":"xyz"}'
And this is my endppoint handler:
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
console.log(req.body)
return res.status(200).end()
}
This the log result:
[Object: null prototype] { "'{username:xyz,password:xyz}'": '' }
Why does it treat the entire data as a key value??
How am I suppose to parse it correctly?
curl -X POST http://localhost:3000/api/test -H 'Content-Type:application/json' --data '{"username":"xyz","password":"xyz"}'
And this is my endppoint handler:
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
console.log(req.body)
return res.status(200).end()
}
This the log result:
[Object: null prototype] { "'{username:xyz,password:xyz}'": '' }
Why does it treat the entire data as a key value??
How am I suppose to parse it correctly?
Answered by not-milo.tsx
Windows cmd doesn't support single quotes... Replace them with double quotes and escape any json double quotes with back slashes like so:
curl -d "{\"key1\":\"value1\", \"key2\":\"value2\"}" -H "Content-Type: application/json" -X POST http://localhost:3000/api/webhook
51 Replies
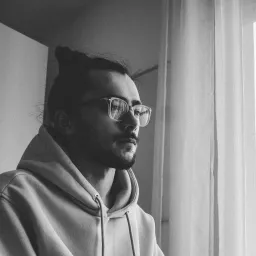
You need to parse the body like this:
const body = JSON.parse(req.body);
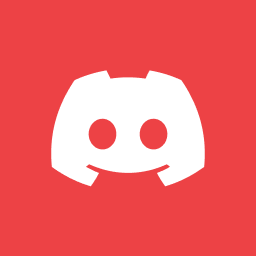
Atlantic herringOP
I got error - TypeError: Cannot convert object to primitive value
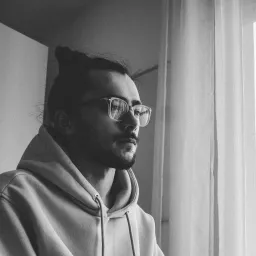
Try using this command and see if the outcome is the same:
curl -d '{"key1":"value1", "key2":"value2"}' -H "Content-Type: application/json" -X POST http://localhost:3000/api/test
I'm not sure, but you might have to use double quotes when specifying the content tipe instead of single quotes.
So instead of
So instead of
-H 'Content-Type: application/json'
try using -H "Content-Type: application/json"
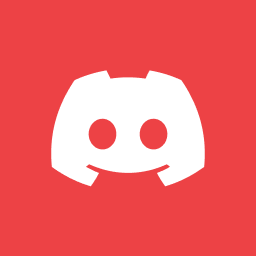
Atlantic herringOP
No single quotes doesnt work, INVALID JSON
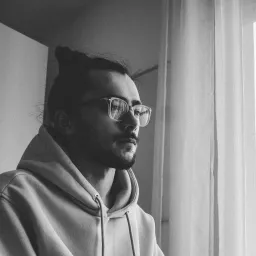
Can you make a minimum reproduction repository so I can try it and see what's going on?
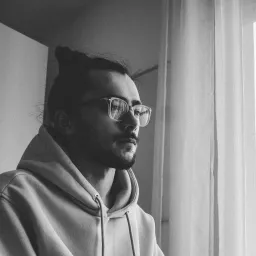
And this throws the same error for you?
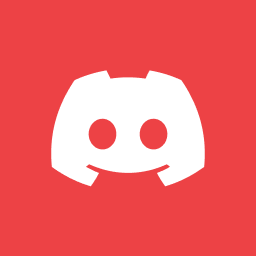
Atlantic herringOP
Yes
Not to you?
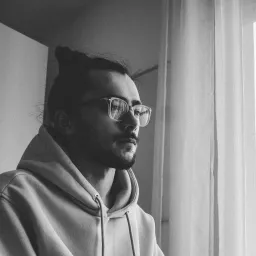
I just tried it and it works as expected
Can you try making the request with a tool like Postman?
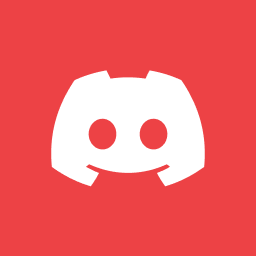
Atlantic herringOP
Yes I already tried with Insomia and it works as expected. But with curl it does not. This endpoint is a webhook which is called by another service and I got the same error of calling it by curl
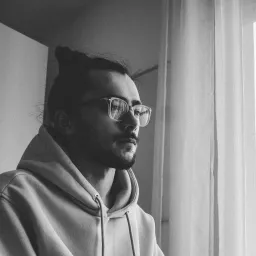
It could be an issue with curl
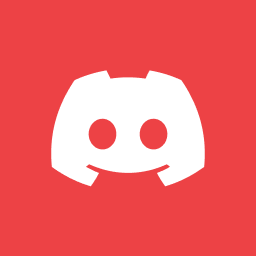
Atlantic herringOP
What is your output of the console.log func?
I thought the same until it had failed with the other service request
Thus it cannot be a curl only problem,
Are you on Windows by any chance?
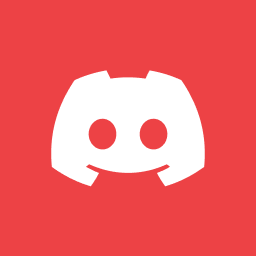
Atlantic herringOP
Yes windows 11

This is what happends with single quote

Curl fails
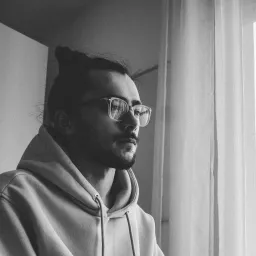
Windows cmd doesn't support single quotes... Replace them with double quotes and escape any json double quotes with back slashes like so:
curl -d "{\"key1\":\"value1\", \"key2\":\"value2\"}" -H "Content-Type: application/json" -X POST http://localhost:3000/api/webhook
Answer
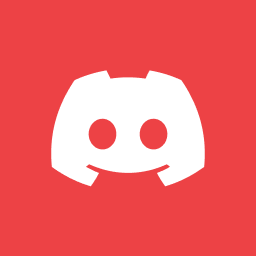
Atlantic herringOP

Still no way...
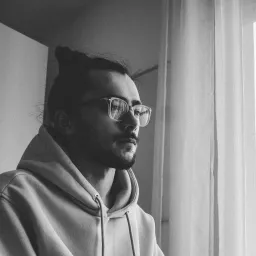
Sorry, I meant back slashes
I edited the example above. Try copying it again and let me know if it works
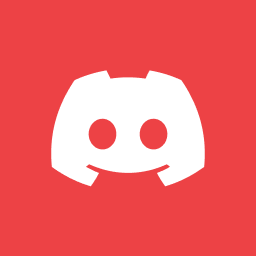
Atlantic herringOP
Ah ok now it worked!
Still have problems with a service, but cannot understand whyh
Thank u btw
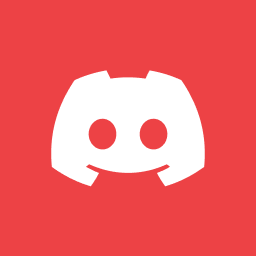
Atlantic herringOP
Yes
TradingView
Anyway would u know how to parse the body also for my case? Since I cannot chenge the post request of the service (TradingView)
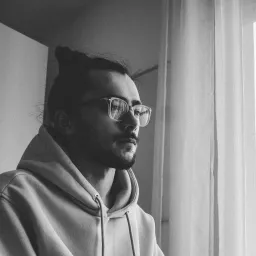
If this is how your data is received 100% of the time you can try getting the string representation of the body an manipulate that to extract the actual data from the malformed key. Then use
JSON.parse()
on the result and you have your data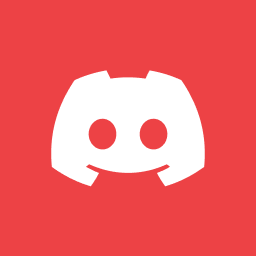
Atlantic herringOP
Ok but how can I get the string value I need of this object:
[Object: null prototype] { "'{username:xyz,password:xyz}'": '' }
req.body[0] returns
undefined
But why does this problem happend?
Why? You too?
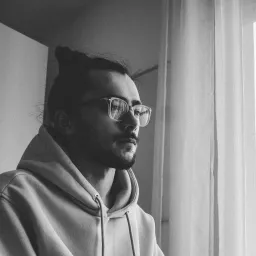
I don't know how TradingView works, but it's probably running into the same issue with curl that you had...
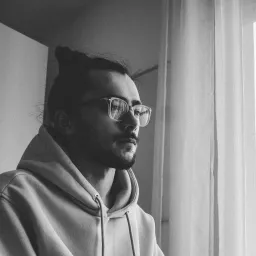
I've added an example of this directly in a PR of your repo. I'll add it here for reference should someone else need it.
In your API route export this configuration object as documented [here](https://nextjs.org/docs/pages/building-your-application/routing/api-routes#custom-config):
Then inside the handler use the [
Once you have your raw body, you can do whatever you need to do with it.
In your API route export this configuration object as documented [here](https://nextjs.org/docs/pages/building-your-application/routing/api-routes#custom-config):
export const config = {
api: {
bodyParser: false,
},
};
Then inside the handler use the [
raw-body
](https://www.npmjs.com/package/raw-body) and [content-type
](https://www.npmjs.com/package/content-type) packages to get a string representation of the request body like so:import type { NextApiRequest, NextApiResponse } from "next";
import contentType from "content-type";
import getRawBody from "raw-body";
export default async function handler(
req: NextApiRequest,
res: NextApiResponse
) {
getRawBody(
req,
{
length: req.headers["content-length"],
limit: "1mb",
encoding: contentType.parse(req).parameters.charset,
},
function (err, rawBody) {
if (err) return res.status(500).send(err);
console.log(rawBody.toString()); // Do what you need with the raw body
}
);
return res.status(200).json("Done!");
}
Once you have your raw body, you can do whatever you need to do with it.
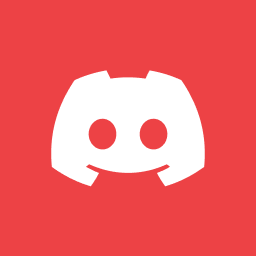
Atlantic herringOP
Awesome I didn t knwo how to remove the auto json parser from it and know it's working!
Molto belli comunque i tuoi proggetti!
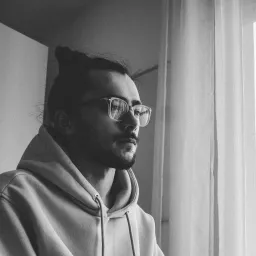
Great. The rest is up to you to solve ✌ðŸ»
Grazie! ✨