Forwarding To Checkout after Signing in with Supabase:
Answered
Ancient Murrelet posted this in #help-forum
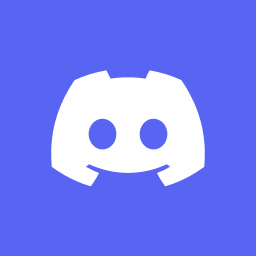
Ancient MurreletOP
I’m trying to forward users to checkout if they don’t have u subscription after signin, does this code looks fine to you guys?
import {
getActiveProductsWithPrices,
getSession,
getSubscription,
} from '@/app/supabase-server';
import AuthUI from './AuthUI';
import { redirect } from 'next/navigation';
import Redirect from '@/components/Redirect';
export default async function SignIn() {
const [session, subscription, products] = await Promise.all([getSession(), getSubscription(), getActiveProductsWithPrices()]);
if (session && subscription === null) {
return <Redirect products={products} />
}
if (session) {
return redirect('/account');
}
return (
...Auth
);
}
and then the Redirect Component:
'use client';
import { postData } from '@/utils/helpers';
import { getStripe } from '@/utils/stripe-client';
import { useEffect } from 'react';
import LoadingScreen from '@/components/LoadingScreen';
export default function Redirect({products }) {
useEffect(() => {
const getCheckout = async () => {
const price = products[0].prices[0];
const { sessionId } = await postData({
url: '/api/create-checkout-session',
data: { price }
});
const stripe = await getStripe();
stripe?.redirectToCheckout({ sessionId });
}
getCheckout();
},[products]);
return <LoadingScreen />;
}
It works, but it just seems a bit slow to happen……
import {
getActiveProductsWithPrices,
getSession,
getSubscription,
} from '@/app/supabase-server';
import AuthUI from './AuthUI';
import { redirect } from 'next/navigation';
import Redirect from '@/components/Redirect';
export default async function SignIn() {
const [session, subscription, products] = await Promise.all([getSession(), getSubscription(), getActiveProductsWithPrices()]);
if (session && subscription === null) {
return <Redirect products={products} />
}
if (session) {
return redirect('/account');
}
return (
...Auth
);
}
and then the Redirect Component:
'use client';
import { postData } from '@/utils/helpers';
import { getStripe } from '@/utils/stripe-client';
import { useEffect } from 'react';
import LoadingScreen from '@/components/LoadingScreen';
export default function Redirect({products }) {
useEffect(() => {
const getCheckout = async () => {
const price = products[0].prices[0];
const { sessionId } = await postData({
url: '/api/create-checkout-session',
data: { price }
});
const stripe = await getStripe();
stripe?.redirectToCheckout({ sessionId });
}
getCheckout();
},[products]);
return <LoadingScreen />;
}
It works, but it just seems a bit slow to happen……
Answered by Ancient Murrelet
I think a server-side-redirect is more efficient I’ve done this solution:
import {
getActiveProductsWithPrices,
getSession,
getSubscription,
} from '@/app/supabase-server';
import AuthUI from './AuthUI';
import { redirect } from 'next/navigation';
import { stripe } from '@/utils/stripe';
export default async function SignIn(req: any, res: any) {
const [session, subscription, products] = await Promise.all([getSession(), getSubscription(), getActiveProductsWithPrices()]);
if (session && subscription == null) {
console.log(req)
const session = await stripe.checkout.sessions.create({
line_items: [
{
price: products[0].prices[0].id,
quantity: 1
}],
mode: 'subscription',
success_url: `http://localhost:3000/account`,
cancel_url: `http://localhost:3000/account`,
})
console.log(session)
return redirect(session.url as string)
}
if (session) {
return redirect('/account');
}
return (
<div className='flex justify-center height-screen-helper'>
<div className='flex flex-col justify-between max-w-lg p-3 m-auto w-80 '>
<div className='flex justify-center pb-12 '>
</div>
<AuthUI />
</div>
</div>
);
}
4 Replies
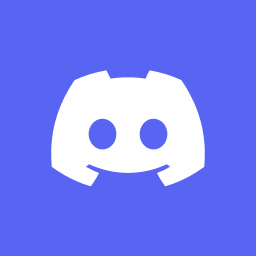
Ancient MurreletOP
I think a server-side-redirect is more efficient I’ve done this solution:
import {
getActiveProductsWithPrices,
getSession,
getSubscription,
} from '@/app/supabase-server';
import AuthUI from './AuthUI';
import { redirect } from 'next/navigation';
import { stripe } from '@/utils/stripe';
export default async function SignIn(req: any, res: any) {
const [session, subscription, products] = await Promise.all([getSession(), getSubscription(), getActiveProductsWithPrices()]);
if (session && subscription == null) {
console.log(req)
const session = await stripe.checkout.sessions.create({
line_items: [
{
price: products[0].prices[0].id,
quantity: 1
}],
mode: 'subscription',
success_url: `http://localhost:3000/account`,
cancel_url: `http://localhost:3000/account`,
})
console.log(session)
return redirect(session.url as string)
}
if (session) {
return redirect('/account');
}
return (
<div className='flex justify-center height-screen-helper'>
<div className='flex flex-col justify-between max-w-lg p-3 m-auto w-80 '>
<div className='flex justify-center pb-12 '>
</div>
<AuthUI />
</div>
</div>
);
}
Answer
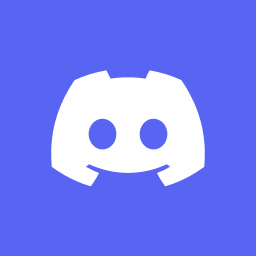
Ancient MurreletOP
@joulev thank you, did you read over it as well? or just assumed I got it right?

joulev
the code is not correct entirely. server components don't have
req: any, res: any
. but the idea of server-side redirect is correct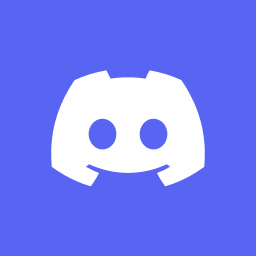
Ancient MurreletOP
@joulev Yes I fixed that by now. Thank you, were just small artifacts of finding the correct solution.