How to apply styles to specific component in next js.
Unanswered
Iridescent shark posted this in #help-forum
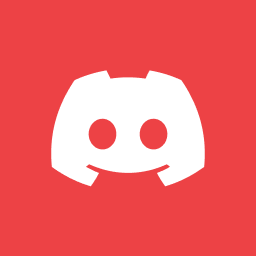
Iridescent sharkOP
I have a component that displays markdown.
Also I have styles in css file which I want to apply only to html content of this component. How I can add them so they are not apply to anything outside of this component? Some of styles are not classes.
"use client"
import React, { useEffect, useState } from 'react'
import './styles.css'
import { markdownToHtml } from '@/lib/helper-utils';
interface MarkDownProps {
markdown: string;
}
const MarkDown: React.FC<MarkDownProps> = ({ markdown }) => {
const [htmlContent, setHtmlContent] = useState<string>();
useEffect(() => {
const processMarkdown = async () => {
const html = await markdownToHtml(markdown);
setHtmlContent(html);
}
processMarkdown();
}, []);
if (!htmlContent) return <><p>Loading content...</p></>
return (
<div dangerouslySetInnerHTML={{ __html: htmlContent }} />
)
}
export default MarkDown
Also I have styles in css file which I want to apply only to html content of this component. How I can add them so they are not apply to anything outside of this component? Some of styles are not classes.
code[class*="language-"],
pre[class*="language-"] {
color: #393A34;
font-family: "Consolas", "Bitstream Vera Sans Mono", "Courier New", Courier, monospace;
direction: ltr;
text-align: left;
white-space: pre;
word-spacing: normal;
word-break: normal;
font-size: .9em;
line-height: 1.2em;
-moz-tab-size: 4;
-o-tab-size: 4;
tab-size: 4;
-webkit-hyphens: none;
-moz-hyphens: none;
-ms-hyphens: none;
hyphens: none;
}
11 Replies

joulev
or
.content code[class*="language-"],
.content pre[class*="language-"] {
whatever...
}
and use
className="content"
in the component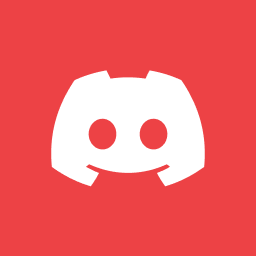
Iridescent sharkOP
I tried to use module css but I says that I can't use styles that applied to html element, only class name
The only working way is to add .class before styles? There are ~ 50 different styles

joulev
oh yeah i forgot about this
look at css nesting solutions, like scss or postcss nesting
if you are using tailwind, [it has first class support of css nesting](https://tailwindcss.com/docs/using-with-preprocessors#nesting)
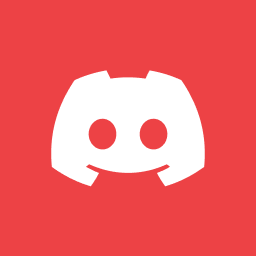
Iridescent sharkOP
Thank you, I tried scss +
+ classname = "markdown_content" and it works fine, but if I try to rename it to module and use as className={styles.markdown_content}, for some reason some styles for child html elements are not applied
.markdown_content {
code[class*="language-"],
...
}
+ classname = "markdown_content" and it works fine, but if I try to rename it to module and use as className={styles.markdown_content}, for some reason some styles for child html elements are not applied

joulev
About the module css part, what do you mean by “some styles for child html elements are not applied�
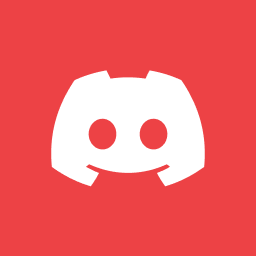
Iridescent sharkOP
thank you but I considered to switch to mdx which is more easy to setup