Pattern to pass dynamic info from a client component to a child server component
Unanswered
Californian posted this in #help-forum
CalifornianOP
I have a client component where cookies are set when clicking on buttons (with react-cookie lib). It also receives a server component as props. Something like:
The child server component access the cookies using Next.js cookies() and read and display some products from the db. Something like:
Issue: when I refresh the app, I can alternate pages for a very short while by clicking on the buttons. But after this while, I can only alternate pages again if I refresh the page. Any idea ?
"use client"
import { useRouter } from 'next/navigation';
import { useCookies } from 'react-cookie';
export default function Panel({
children,
}: {
children: React.ReactNode
}) {
const router = useRouter();
const [cookies, setCookie] = useCookies(['pageNumber']);
return (
<div>
<ul>
<li>
<button onClick={() => {
setCookie('pageNumber', 1, { path: '/' });
router.replace("/");
}}>Page 1</button>
</li>
<li>
<button onClick={() => {
setCookie('pageNumber', 2, { path: '/' });
router.replace("/");
}}>Page 2</button>
</li>
</ul>
{children}
</div>
);
}
The child server component access the cookies using Next.js cookies() and read and display some products from the db. Something like:
import { cookies } from 'next/headers';
import getProducts from '@/components/catalog/utils';
export default async function Products() {
const pageNumber = Number(cookies().get('pageNumber')?.value);
if (pageNumber) {
const products = await getProducts(pageNumber);
return (
<div>
<ul>
{products.map((product) => (
<li key={product.id}>
<h2>{product.name}</h2>
</li>
))}
</ul>
</div>
);
}
}
Issue: when I refresh the app, I can alternate pages for a very short while by clicking on the buttons. But after this while, I can only alternate pages again if I refresh the page. Any idea ?
4 Replies
CalifornianOP
Also, if someone has a better pattern to pass dynamic info from a client component to a child server component, or any critics on this structure, I would like to hear.
CalifornianOP
I tried using the Link component instead of the useRouter hook but it gaves the same effect...
CalifornianOP
Using the refresh method of the router object instead of the replace method makes it work
But probably not the best way. I am still interested in what patterns you guys use to do this
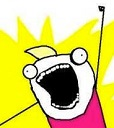
But probably not the best way. I am still interested in what patterns you guys use to do this
@Californian Using the refresh method of the router object instead of the replace method makes it work <:all_the_things:753872185118162965>
But probably not the best way. I am still interested in what patterns you guys use to do this
if you want full refreshes each click you need to use a standard <a> or window.location.href