Infinite fetch loop on development server
Unanswered
Mini Satin posted this in #help-forum
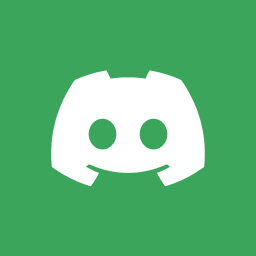
Mini SatinOP
I have a page that calls a server actions that does a fetch o a different route. While the route is compiling, it got like an infinite loop of requests. I think it only happens on the development server
This is my page
and this is the server action that makes the fetch operation
This is my page
import { startCheckoutServerAction } from "@/app/checkout/actions/startCheckoutServerAction";
import StartOrder from "@/app/checkout/components/StartOrder";
import { searchParamsToQueryString } from "@/utils/pages/searchParams";
export default async function Page({
searchParams,
}: {
searchParams: { [key: string]: string | string[] | undefined };
}) {
const checkout = await startCheckoutServerAction(searchParamsToQueryString(searchParams));
return <StartOrder checkout={checkout} />;
}
and this is the server action that makes the fetch operation
"use server";
export async function startCheckoutServerAction(queryString: string): Promise<StartCheckoutResponse> {
const response = await fetch(`${currentBaseUrl()}/api/checkout/2023-07-17/start?${queryString}`, {
cache: "no-cache",
});
return await response.json();
}
3 Replies
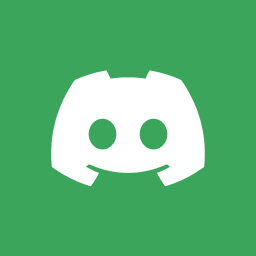
Mini SatinOP
This is from the web perspective

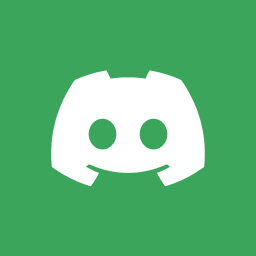
European sprat
Show StartOrder component
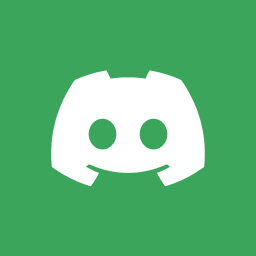
Mini SatinOP
"use client";
import { setInitCookieAction } from "@/app/checkout/actions/setInitCookieAction";
import CheckoutLoading from "@/app/checkout/components/LoadingComponent";
import { useRouter } from "next/navigation";
import { useEffectOnce } from "usehooks-ts";
import {
StartCheckoutErrorResponse,
StartCheckoutResponse,
StartCheckoutSuccessResponse,
} from "@/types/Checkout/startCheckout";
export default function StartOrder({ checkout }: { checkout: StartCheckoutResponse }) {
const router = useRouter();
useEffectOnce(() => {
if ((checkout as StartCheckoutSuccessResponse).orderIdentifier != null) {
const success = checkout as StartCheckoutSuccessResponse;
setInitCookieAction(success).then(() =>
router.push(`/checkout/order/${(checkout as StartCheckoutSuccessResponse).orderIdentifier}`)
);
} else {
const errorResponse = checkout as StartCheckoutErrorResponse;
router.push(`/error?error=${JSON.stringify(errorResponse.errorStatus)}`);
}
});
return <CheckoutLoading includeLayout={true} />;
}
and setInitCookieAction
"use server";
import { cookies } from "next/headers";
import { StartCheckoutSuccessResponse } from "@/types/Checkout/startCheckout";
export async function setInitCookieAction(response: StartCheckoutSuccessResponse) {
const orderIdentifier = response.orderIdentifier;
const clientSecret = response.clientSecret;
cookies().set(`${orderIdentifier}.clientSecret`, clientSecret, {
path: "/",
secure: true,
expires: new Date(Date.now() + 60 * 60 * 1000 * 24),
});
}