Streaming data with <Suspense>
Unanswered
Red-naped Sapsucker posted this in #help-forum
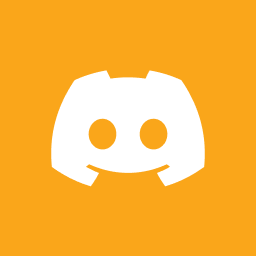
Red-naped SapsuckerOP
Ok, so I have a page that displays a number of articles. These articles have a property named FOO. The value of FOO for most articles can be fetched together with the articles themselves, but for some articles, a separate, long running API call has to be made. I would like to display all the articles as soon as they're available, and populate the value of FOO on the articles once it has been retrieved. I read about streaming using
<Suspense>
but am not sure if that will work for what I'm trying to do?2 Replies
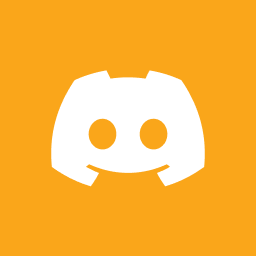
Red-naped SapsuckerOP
Ok i just played around with it and this seems to work:
import { Suspense } from "react";
export default async function StreamingTest() {
return <main>
<Article description="Immediately available" delayed={false} />
<Article description="" delayed={true} />
</main>;
}
function Article({ description, delayed }: { description: string, delayed: boolean }) {
if (!delayed) {
return <Description description={description} />;
} else {
return <Suspense fallback={<Description description="..." />}><DelayedDescription /></Suspense>;
}
}
function Description({ description }: { description: string }) {
return <div>Description: {description}</div>;
}
async function DelayedDescription() {
const description = await getDelayedDescription();
return <Description description={description} />;
}
async function getDelayedDescription(): Promise<string> {
return new Promise( resolve => {
setTimeout( () => resolve( "Only available after 2 seconds" ), 2000 );
} );
}
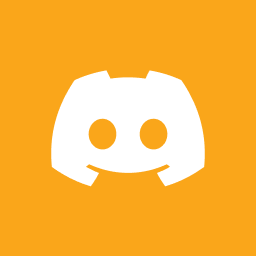
Red-naped SapsuckerOP
Is there any way I can get rid of that extra component to wrap the call to
getDelayedDescription
?