How to do server fetching based on client state?
Unanswered
Cuban Crocodile posted this in #help-forum
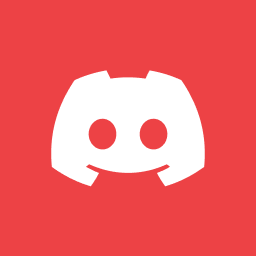
Cuban CrocodileOP
I have a select-component where I am populating the select-items based off of a fetch call on useEffect. I want to do server-component fetching based on this value. What is the best way to go about this from an architectural standpoint using Next?
Currently, I am setting the url-params based on the select value and fetching off-of that. This however, makes my server components return null when the select is being fetched.
Any advice?
Currently, I am setting the url-params based on the select value and fetching off-of that. This however, makes my server components return null when the select is being fetched.
Any advice?
7 Replies

fuma 💙 joulev
You can make the Select component a client component, then pass data from a server component.
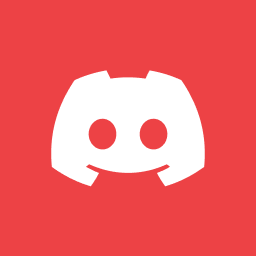
Cuban CrocodileOP
Passing through props, right? How do I go about re-validating the result from the server components on select?

fuma 💙 joulev
Revalidating the data from server side? You can do it using the
revalidate
function or configuring revalidate options of fetch
.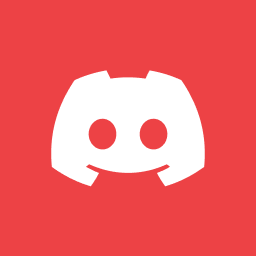
Cuban CrocodileOP
What I am confused with, is how I update the content dynamically based on the select. So when I select customer 1, I fetch data based on customer 1, as an example.

fuma 💙 joulev
In this case, it's better to fetch the data on the client side.
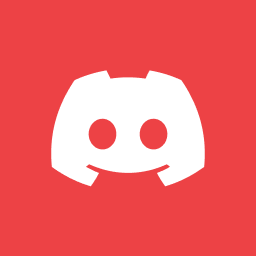
Cuban CrocodileOP
Okay, cool. I figured as well. The best way to go about this is still with useEffect as I understand, it just seems so clunky now that we have server components.
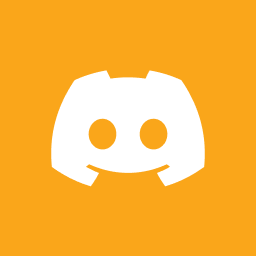
Siberian Flycatcher
What I am confused with, is how I update the content dynamically based on the select. So when I select customer 1, I fetch data based on customer 1, as an example.
You can move the state to the URL and fetch everything in the server component.
[app/[id]/page.jsx]
async function Page({ params }) {
// Get customer list for select
const customerList = await fetch("myapi/customer-lit");
const { id } = params;
// Get currently selected customer baed on params
const selectedCustomer = await fetch(`myapi/customers/${id}`);
return (
<>
{/** Selected customer info */}
{selectedCustomer.name}
{/** ... */}
<SelectCustomer>
{customerList.map((customer) => (
<option value={customer.id}>{customer.name}</option>
))}
</SelectCustomer>
</>
);
}
[app/[id]/SelectCustomer.jsx]
"use client"
import { router } from "next/navigation";
function SelectCustomer({ children }) {
const router = useRouter();
return (
<select onChange={(e) => router.push(`/${e.target.value}`)}>
{children}
</select>
);
}