How to style navbar buttons depending on what page is selected?
Unanswered
EXPANDdoηg posted this in #help-forum

Hi, im new to using next.js
I have a navbar component in my layout file and i want to style the button of the corresponding page when its selected, GPT-4 told me to use useRouter but since it has older data i couldnt get a good answer.
how would i go about doing this?
Is my navbar component ignore the duplicate paths i havent made all the pages yet
is the NavLink component used
Incase its needed here is the layout file:
I have a navbar component in my layout file and i want to style the button of the corresponding page when its selected, GPT-4 told me to use useRouter but since it has older data i couldnt get a good answer.
how would i go about doing this?
"use client"
import { useRouter } from 'next/navigation'
import NavLink from 'components/NavLink'
const Nav = ({children}) => {
const router = useRouter()
return (
<nav className='bg-slate-200 w-full h-20 flex flex-row px-4 py-2 items-center justify-center gap-4'>
<NavLink href={'/chats/personal'} router={router}>Favorites</NavLink>
<NavLink href={'/chats/personal'} router={'personal'}>Messages</NavLink>
<NavLink href={'/chats/networks'} router={'networks'}>Networks</NavLink>
<NavLink href={'/chats/personal'} router={router}>Settings</NavLink>
</nav>
)
}
export default Nav
Is my navbar component ignore the duplicate paths i havent made all the pages yet
import Link from 'next/link'
const NavLink = ({ children, href, router }) => {
let className = router.pathname === href ? 'text-red-500' : 'text-black'
return (
<Link href={href} className={className}>
{children}
</Link>
)
}
export default NavLink
is the NavLink component used
Incase its needed here is the layout file:
import '../globals.css'
import { Inter } from 'next/font/google'
import Nav from 'components/Nav'
export const metadata = {
title: 'My Chats',
description: 'Generated by create next app',
}
export default function RootLayout({
children,
}: {
children: React.ReactNode
}) {
return (
<html lang="en" className='min-h-screen'>
<body className="h-full w-full">
<Nav />
<main className="h-full w-full">
{children}
</main>
</body>
</html>
)
}
5 Replies
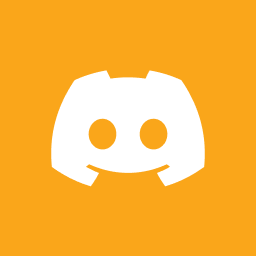
Black-crowned Night-Heron
you might want to check this out. You want to check your active link, and then do customization.
https://nextjs.org/docs/app/building-your-application/routing/linking-and-navigating#checking-active-links
https://nextjs.org/docs/app/building-your-application/routing/linking-and-navigating#checking-active-links

okay thank you ill try that
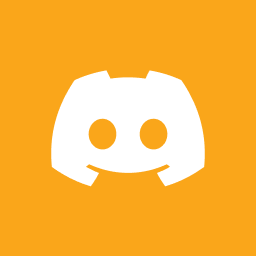
Black-crowned Night-Heron
here is a quick Nav that I have. This changes the
loginMenu
button. You might not have to check active link const logInMenu = (user, logoutUser, menuType) => {
if (menuType === "Main") {
return (
<>
{user ? (
<ActionIcon onClick={logoutUser}>
<IoLogOut className="icon" />
</ActionIcon>
) : (
<ActionIcon component="a" href="/signin">
<BsFillPersonFill className="icon" />
</ActionIcon>
)}
</>
);
} else {
return (
<>
{" "}
{user ? (
<Button onClick={logoutUser} leftIcon={<IoLogOut className="icon" />}>
<span>Log Out</span>
</Button>
) : (
<Button
leftIcon={<BsFillPersonFill className="icon" />}
component="a"
href="/signin"
>
<span>Log In</span>
</Button>
)}
</>
);
}
};
const menuItems = [
{ href: "/home", text: "Home", prefetch: false },
{ href: "/greektime", text: "Time", prefetch: false },
{ href: "/backtest", text: "Back Test", prefetch: false },
{ href: "/about", text: "About", prefetch: true },
];
const HeaderMenu = () => {
const [drawerOpened, { toggle: toggleDrawer, close: closeDrawer }] =
useDisclosure(false);
const [opened, { open, close }] = useDisclosure(false);
const { classes, theme } = useStyles();
const { user, logoutUser } = useFBAuth();
function links() {
return (
<>
<ThemeToggle />
{menuItems.map((link) => (
<Link
href={link.href}
className={classes.link}
prefetch={link.prefetch}
key={`${link.href}+${link.text}`}
>
{link.text}
</Link>
))}
<Button onClick={open} variant="white">
Contact
</Button>
<ModalComp opened={opened} open={open} close={close}>
<Contact />
</ModalComp>
<Link href="/music" className={classes.link}>
Music
</Link>
{/* <ThemeToggler></ThemeToggler> */}
</>
);
}
return (
<>
<Header className={classes.header} height="">
{links}
{logInMenu(user, logoutUser, "")}
</>
)
}

how am i supposed to use usePathname()?