Server Component isnt on the server
Answered
Gazami crab posted this in #help-forum
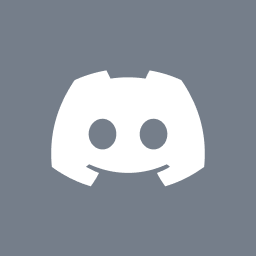
Gazami crabOP
I have this "server component" that renders the sidebar. its inside a client component that has the context, and it renders multiple client components, however when i do console.log, the output shows up in the browser console. How would i fix that?
import { HomeIcon, ListVideoIcon, SettingsIcon } from "lucide-react";
import Logo from "../(site)/Logo";
import Group from "./Group";
import Item from "./Item";
export default function Sidebar() {
console.log("server component");
return (
<nav className="sticky top-0 h-screen w-60 pt-8">
<div className="justify-center-center mb-8 flex w-full">
<Logo />
</div>
<Item name="Home" href="/dashboard">
<HomeIcon />
</Item>
<Group
name="Demos"
subOptions={[
{ name: "New Demos", href: "/dashboard/selection/new" },
{ name: "Demo Stream", href: "/dashboard/selection/stream" },
{ name: "Highlights", href: "/dashboard/selection/highlights" },
{ name: "Discarded", href: "/dashboard/selection/discarded" },
]}
>
<ListVideoIcon />
</Group>
<Item name="Settings" href="/dashboard/settings">
<SettingsIcon />
</Item>
</nav>
);
}
Answered by Gazami crab
Thanks, this is how I ended up resolving the issue:
//layout.tsx
import React from "react";
import RootLayout from "./Nav";
import Sidebar from "./sidebar";
export default function ParentComponent({
children,
}: {
children: React.ReactNode;
}) {
return <RootLayout sidebar={<Sidebar />}>{children}</RootLayout>;
}
2 Replies

joulev
the supposedly server component is imported to a client component, which makes that "server" component actually a client component
you have to follow this pattern to "use" server components inside client components https://nextjs.org/docs/getting-started/react-essentials#nesting-server-components-inside-client-components
"use client" sits between server-only and client code. It's placed at the top of a file, above imports, to define the cut-off point where it crosses the boundary from the server-only to the client part. Once "use client" is defined in a file, all other modules imported into it, including child components, are considered part of the client bundle.
Since Server Components are the default, all components are part of the Server Component module graph unless defined or imported in a module that starts with the "use client" directive. – https://nextjs.org/docs/getting-started/react-essentials#the-use-client-directive
you have to follow this pattern to "use" server components inside client components https://nextjs.org/docs/getting-started/react-essentials#nesting-server-components-inside-client-components
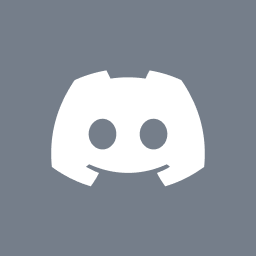
Gazami crabOP
Thanks, this is how I ended up resolving the issue:
//layout.tsx
import React from "react";
import RootLayout from "./Nav";
import Sidebar from "./sidebar";
export default function ParentComponent({
children,
}: {
children: React.ReactNode;
}) {
return <RootLayout sidebar={<Sidebar />}>{children}</RootLayout>;
}
Answer